Here is a way to really adapt the open file dialog for 64 bit.
The only issue is that you can't use the initial directory parameter due to the fact that it is through a PowerShell call. (Fixed this as I was writing this post.)
A screenshot of the dialog:
Here is the PowerShell code, fully fixed to accept a custom title, Start Directory, and Filter for filetypes
$PSDefaultParameterValues['Out-File:Encoding'] = 'utf8'
Add-Type -AssemblyName System.Windows.Forms
$FileBrowser = New-Object System.Windows.Forms.OpenFileDialog -Property @{
}
$FileBrowser.Title = $args[0]
$initial = $args[1].ToString().Replace("/","\")
$FileBrowser.InitialDirectory = $initial
$chosenfilter = $args[2].ToString().Replace("^/^","|")
$FileBrowser.Filter = $chosenfilter
$null = $FileBrowser.ShowDialog()
$FileName = $FileBrowser.FileName
if ($FileName -ne ''){
$FileName > 'openfilename.txt'
exit 1
}
else{
exit 0
}
And here is the current QB64 code that would allow you to call a function similar to GetOpenFileNameA and display an Open File Dialog:
OFile$ = GetOpenFileName64("Select a file, jackass", "C:\Users\zacharys\[redacted]\Desktop\QB64 Files\qb32", "QB64 Files (*.BAS)|*.bas|All Files (*.*)|*.*")
FUNCTION GetOpenFileName64$
(Title$
, InitialDir$
, Filter$
) SHELL$
= "PowerShell -WindowStyle Hidden -ExecutionPolicy Bypass " + CHR$(34) + "&'" + _STARTDIR$ + "\OpenFile.ps1'" InitialDir$ = ReplaceStringItem(InitialDir$, "\", "/")
Filter$ = ReplaceStringItem(Filter$, "|", "^/^")
'Filter$ = ReplaceStringItem(Filter$, "/", "|")
'_ECHO SHELL$
GetOpenFileName64 = OFile$
GetOpenFileName64 = ""
' PRINT "Cancelled"
FUNCTION ReplaceStringItem$
(text$
, old$
, new$
) find
= INSTR(start
+ 1, text$
, old$
) 'find location of a word in text first$
= LEFT$(text$
, find
- 1) 'text before word including spaces last$
= RIGHT$(text$
, LEN(text$
) - (find
+ LEN(old$
) - 1)) 'text after word text$ = first$ + new$ + last$
start = find
ReplaceStringItem = text$
UPDATE!!!!!
2:50 PM: 8:20 AM 5/14/2020
Here is the code for making the GetSaveFileName function:
PowerShell:
$PSDefaultParameterValues['Out-File:Encoding'] = 'utf8'
Add-Type -AssemblyName System.Windows.Forms
$FileBrowser = New-Object System.Windows.Forms.SaveFileDialog -Property @{
}
$FileBrowser.Title = $args[0]
$initial = $args[1].ToString().Replace("/","\")
$FileBrowser.InitialDirectory = $initial
$chosenfilter = $args[2].ToString().Replace("^/^","|")
$FileBrowser.Filter = $chosenfilter
$null = $FileBrowser.ShowDialog()
$FileName = $FileBrowser.FileName
if ($FileName -ne ''){
$FileName > 'savefilename.txt'
exit 1
}
else{
exit 0
}
And QB64:
SFile$ = GetSaveFileName64("Save a file, jackass", "C:\Users\zacharys\[redacted]\Desktop\QB64 Files\qb32", "QB64 Files (*.BAS;*.BI;*.BM;*.FRM)|*.BAS;*.BI;*.BM;*.FRM|All Files (*.*)|*.*")
FUNCTION GetSaveFileName64$
(Title$
, InitialDir$
, Filter$
) SHELL$
= "PowerShell -ExecutionPolicy Bypass " + CHR$(34) + "&'" + _STARTDIR$ + "\SaveFile.ps1'" InitialDir$ = ReplaceStringItem(InitialDir$, "\", "/")
Filter$ = ReplaceStringItem(Filter$, "|", "^/^")
'Filter$ = ReplaceStringItem(Filter$, "/", "|")
'a = SHELL(SHELL$)
'_ECHO SHELL$
GetSaveFileName64 = SFile$
GetSaveFileName64 = ""
' PRINT "Cancelled"
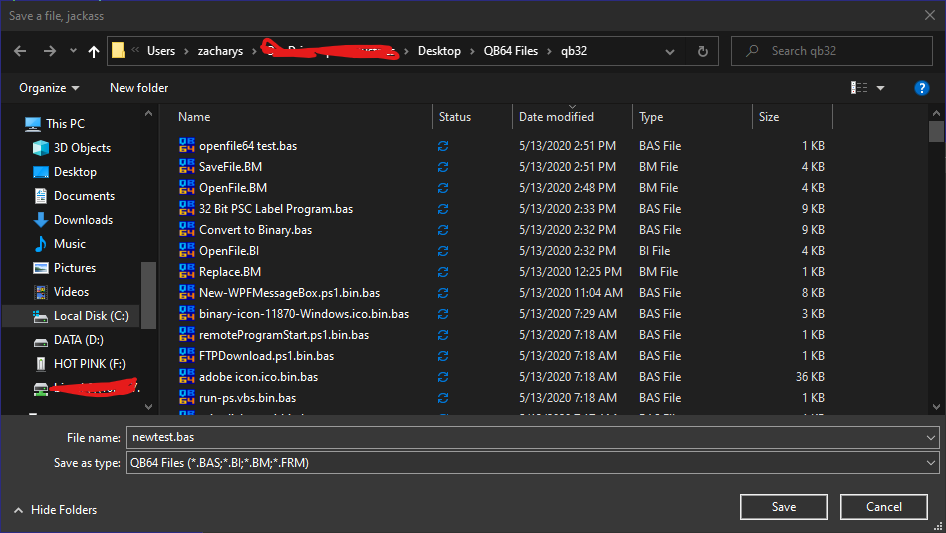